Here are some efficient methods for structuring Bash scripts for Linux environments.
Use Proper Syntax and Structure
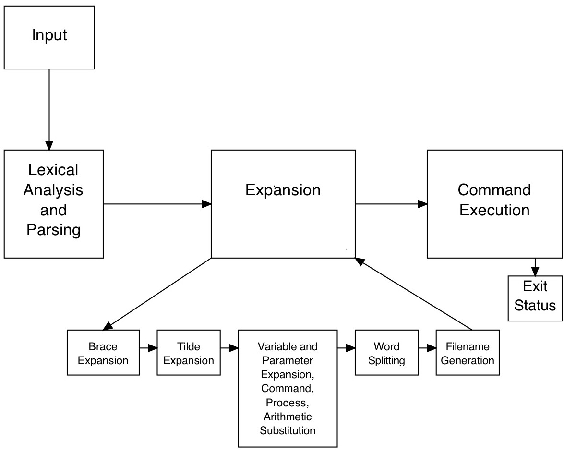
- Start scripts with
#!/usr/bin/env bash
for better portability[2]. - Use
.sh
or.bash
extensions for script files to improve clarity[3]. - Place all code within functions, with a
main
function as the entry point[1].
Optimize Variable Usage
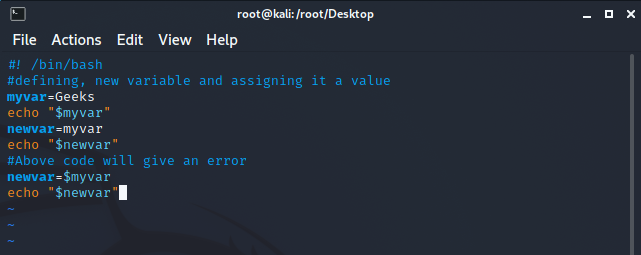
- Use lowercase for variable names unless they’re exported to the environment[1].
- Always use
local
when setting variables within functions[1]. - Surround variables with curly braces:
${variable}
for clarity and to avoid ambiguity[2].
Implement Error Handling and Debugging
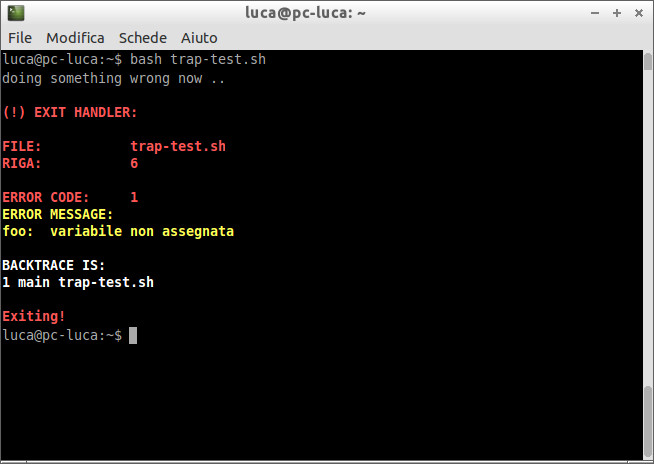
- Use
set -o errexit
(orset -e
) to exit when a command fails[2]. - Implement
set -o nounset
(orset -u
) to catch undeclared variables[2]. - Add
set -o xtrace
(orset -x
) for debugging, controlled by a$TRACE
variable[3].
Improve Script Robustness
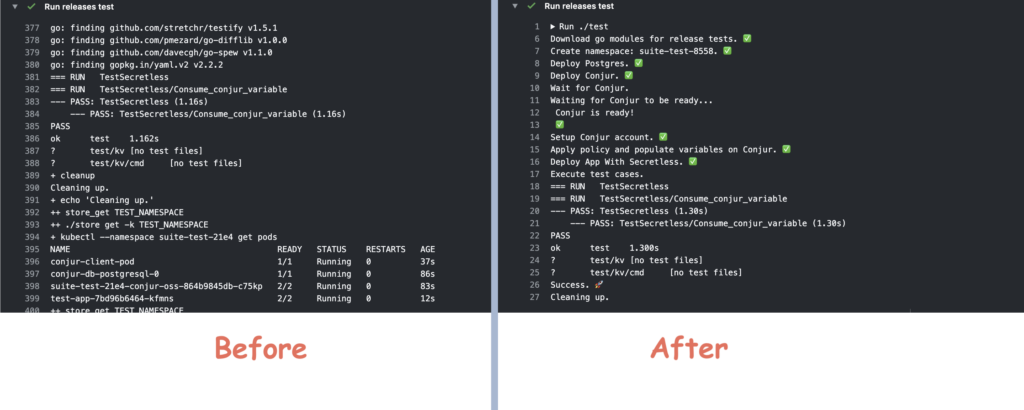
- Use
set -o pipefail
to catch errors in piped commands[2]. - Always quote variable expansions:
"${variable}"
[1][3]. - Use
[[ ]]
for conditionals instead of[ ]
ortest
[3].
Enhance Readability and Maintainability
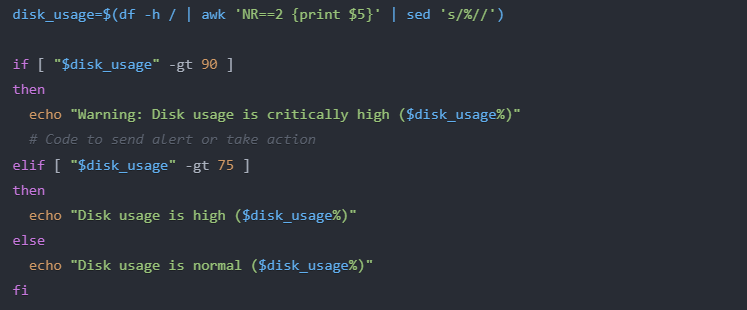
- Use long options for commands (e.g.,
logger --priority
instead oflogger -p
) for better readability[2]. - Add a help option that responds to
-h
,--help
,help
, etc.[3]. - Use descriptive variable and function names[6].
Optimize Performance
- Prefer Bash built-ins over external commands when possible[1].
- Use
printf
instead ofecho
for more powerful and consistent output[1].
Handle Input and Output Properly
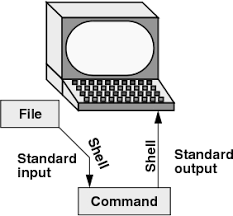
- Redirect error messages to stderr:
echo "Error message" >&2
[3]. - Use
getopts
orgetopt
for parsing command-line options[4].
Follow Best Practices for Specific Scenarios
- Use
mktemp
for temporary files and set up proper cleanup with traps[1]. - For configuration, source a config file after setting default variables[4].
By following these practices, you can create more efficient, readable, and maintainable Bash scripts. Remember to maintain consistency in your coding style and patterns throughout your scripts.
Citations:
[1] https://faun.pub/best-practices-for-writing-bash-scripts-b72fe4099b0?gi=78e3012cb0b8
[2] https://kvz.io/blog/bash-best-practices.html
[3] https://sharats.me/posts/shell-script-best-practices/
[4] https://stackoverflow.com/questions/78497/design-patterns-or-best-practices-for-shell-scripts
[5] https://dev.to/unfor19/writing-bash-scripts-like-a-pro-part-1-styling-guide-4bin
[6] https://www.reddit.com/r/bash/comments/17fj8x0/my_tips_and_tricks_for_bash_scripting_after/